How to use CKEditor in Livewire Laravel 10, Step by Step Guide
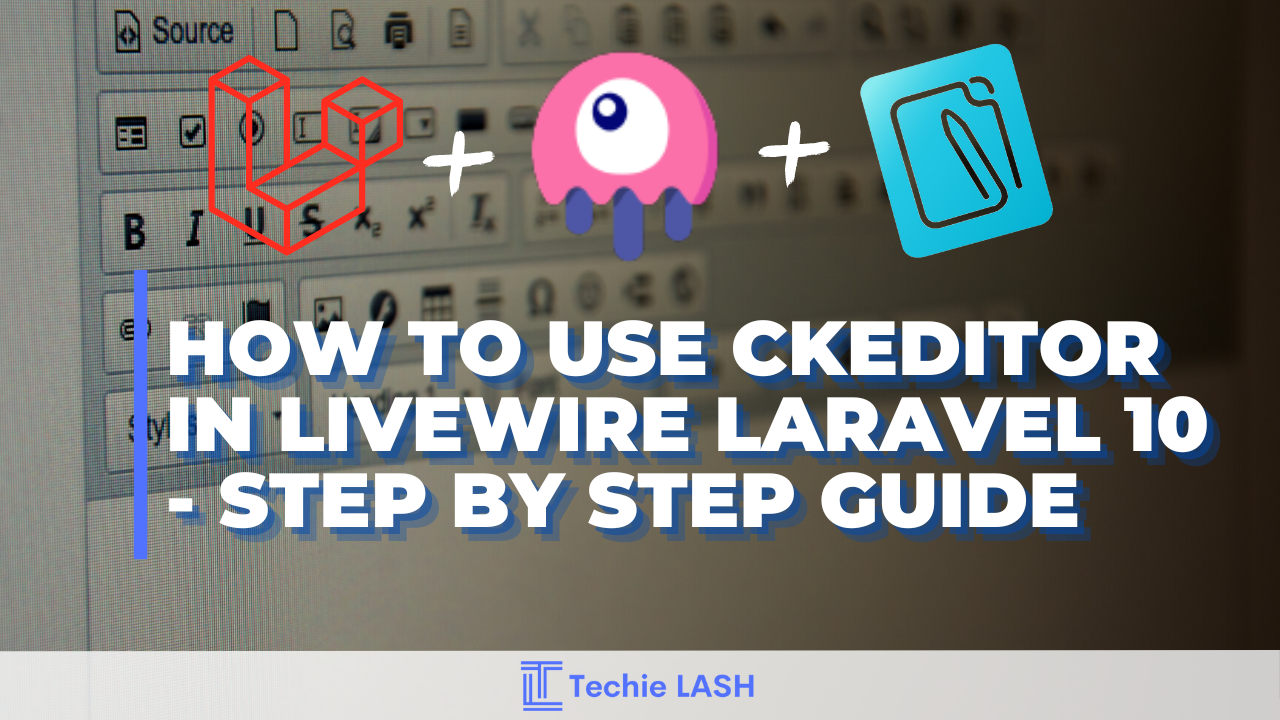
What is CKEditor
CKEditor is a Open Source WYSIWYG HTML editor that can accommodate a variety of use cases, from Word-like documents with expansive toolbars to basic toolbars with a simplified feature set used for emails or instant messaging.
CKEditor provides the environment to use HTML editorable enviroment to your textarea.
Versions of CKEditor
When you go to CKEditor official website you may see there is a two version of CKEditors.
CKEditor 4
CKEditor 4 is like more classic look than the new version of CKEditors.
CKEditor 4 CDN
<script src="https://cdn.ckeditor.com/4.20.2/standard/ckeditor.js"></script>
You can also download the previous versions of the CKEditor 4 CKEditor 4
CKEditor 5
The newest version of CKEditor is the CKEditor 5 it provides simple editor to the enduser.
CKEditor 5 npm package
npm install --save @ckeditor/ckeditor5-build-classic
CKEditor 5 CDN
<script src="https://cdn.ckeditor.com/ckeditor5/36.0.1/classic/ckeditor.js"></script>
You can also download the previous versions of the CKEditor 4 CKEditor 5.
In here you can view demos of CKEditor 5
Types of Editors
- Classic editor - shows a toolbar and boxed editing area that are both positioned specifically on the page.
- Inline editor - allows you to modify the information right in the spot where it will appear, using a floating toolbar that appears when the editable text is focused.
- Balloon editor - provides a balloon toolbar that appears next to the selected editable document element, allowing you to modify material right in its intended location.
- Balloon block editor - allows you to directly generate your content in its intended area with the aid of two toolbars:
- Document editor - The editor is a feature-packed construct designed to provide an experience editing rich content that is comparable to native word processors. It functions best when writing papers that will typically be printed or exported as PDF files later.
How to use CKEditor in your project via CDN
CKEditor 4
First of all you need place CDN link above the closing tag of header element '</head>'
<script src="https://cdn.ckeditor.com/4.20.2/standard/ckeditor.js"></script>
index.html file
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>A Simple Page with CKEditor 4</title>
<script src="https://cdn.ckeditor.com/4.20.2/standard/ckeditor.js"></script>
</head>
<body>
<form>
<textarea name="editor1" id="editor1" rows="10" cols="80">
This is the textarea to be replaced with CKEditor 4.
</textarea>
<script>
// Replace the <textarea id="editor1"> with a CKEditor 4
// instance, using default configuration.
CKEDITOR.replace( 'editor1' );
</script>
</form>
</body>
</html>
CKEditor 4 Sample preview
CKEditor 5
First of all you need place CDN link above the closing tag of header element '</head>'
<script src="https://cdn.ckeditor.com/ckeditor5/36.0.1/classic/ckeditor.js"></script>
index.html file
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>CKEditor 5 – Classic editor</title>
<script src="https://cdn.ckeditor.com/ckeditor5/36.0.1/classic/ckeditor.js"></script>
</head>
<body>
<h1>Classic editor</h1>
<div id="editor">
<p>This is some sample content.</p>
</div>
<script>
ClassicEditor
.create( document.querySelector( '#editor' ) )
.catch( error => {
console.error( error );
} );
</script>
</body>
</html>
CKEditor 5 Sample preview
How to use CKEditor for Your Laravel 10 Livewire Project
Step 1 : Setup the Environment for CKEditor
Make a Laravel layout file called resources/views/layouts/ckeditor.blade.php. We are pulling all of the Javascript and CSS from the relevant CDN network in order to make the tutorial setup simple. To set up the Javascript and CSS locally for your project, however, is highly recommended:
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="csrf-token" content="{{ csrf_token() }}">
<title>Using CKEditor with Laravel Livewire</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css2 family=Nunito:wght@400;600;700&display=swap">
<script src="https://cdn.tailwindcss.com"></script>
<script src="https://cdn.ckeditor.com/ckeditor5/27.1.0/classic/ckeditor.js"></script>
@livewireStyles
</head>
<body class="bg-gray-200" style="font-family: Nunito;">
<div class="max-w-7xl mx-auto px-4 py-4 sm:py-6 lg:py-8 sm:px-6 lg:px-8 ">
{{ $slot }}
</div>
@livewireScripts
@stack('scripts')
</body>
</html>
Step 2 : Create a Livewire Component for CKeditor
To use CKEditor, create a Livewire component. To create the component files, run the CLI command shown below:
php artisan make:livewire Ckeditor
Two created files ought to appear here:
app/Http/Livewire/Ckeditor.php
resources/views/livewire/ckeditor.blade.php
While the view file is located in resources/views/livewire/ckeditor.blade.php, the component class file is located in file app/Http/Livewire/Ckeditor.php.
Let's write a few content for the class file, located at app/Http/Livewire/Ckeditor.php:
<?php
namespace App\Http\Livewire;
use Livewire\Component;
class Ckeditor extends Component
{
public $message;
public function render()
{
return view('livewire.ckeditor')
->layout('layouts.ckeditor');;
}
}
The message entered is stored in the variable $message.
In the function render(), we tell Livewire to use the layout file at resources/views/layouts/ckeditor.blade.php to render a view file at resources/views/livewire/ckeditor.blade.php.
This is the most common method of using the Livewire component.
The CKEditor component of the system will then be worked on, which calls for certain special techniques.
Step 3 : Changing the Livewire View
The content of the view file (resources/views/livewire/ckeditor.blade.php) is below. In the step after you copy and paste it, we'll go over the important sections of the view file:
<div>
<div class="max-w-3xl mx-auto mb-2">
<div class="bg-white rounded-lg">
<div class="max-w-7xl mx-auto py-16 px-4 sm:py-24 sm:px-6 lg:px-8">
<div class="text-center">
<p class="mt-1 text-4xl font-extrabold text-gray-900 sm:text-5xl sm:tracking-tight lg:text-6xl">
Using CKEditor with Laravel Livewire
</p>
</div>
</div>
</div>
</div>
<div class="max-w-3xl mx-auto mb-2">
<div class="bg-white rounded-lg p-5">
<div class="flex flex-col space-y-10">
<div wire:ignore>
<textarea wire:model="message"
class="min-h-fit h-48 "
name="message"
id="message"></textarea>
</div>
<div>
<span class="text-lg">You typed:</span>
<div class="w-full min-h-fit h-48 border border-gray-200">{{ $message }}</div>
</div>
</div>
</div>
</div>
</div>
@push('scripts')
<script>
ClassicEditor
.create(document.querySelector('#message'))
.then(editor => {
editor.model.document.on('change:data', () => {
@this.set('message', editor.getData());
})
})
.catch(error => {
console.error(error);
});
</script>
@endpush
As soon as they are initialized, the DOM is already being altered by the CKEditor, and this process continues while you work with them. We can use Livewire's wire:ignore tag in this situation because Livewire will be unable to keep track of this. It instructs Livewire to disregard this section of the DOM. The code's subsequent section has accomplished the following:
......
<div wire:ignore>
<textarea wire:model="message"
class="min-h-fit h-48 "
name="message"
id="message"></textarea>
</div>
......
Because Livewire has since disregarded the CKEditor section of the code, it is unable to manage the $message variable in its component class.
The text in the textarea is actually all that Livewire needs to know. To set the value of `$message` in a situation like this, we can utilize the unique `$this blade directive`:
@push('scripts')
<script>
ClassicEditor
.create(document.querySelector('#message'))
.then(editor => {
editor.model.document.on('change:data', () => {
@this.set('message', editor.getData());
})
})
.catch(error => {
console.error(error);
});
</script>
@endpush
In the code above, we have accomplished that. We use the `$this` blade directive to set the value for the Livewire variable `$message` after listening for the CKEditor data changing event.
Step 4 : Final Step of Demo
The Livewire component Ckeditor must be rendered to the browser as the final component of the demo, which is easily accomplished using the route file routes/web.php.
Route::get('/ckeditor', Ckeditor::class);
Start a straightforward Laravel server by running npm run dev and php artisan serve, then use your browser to navigate to http://127.0.0.1:8000/ckeditor to view a nice, functional textarea powered by CKEditor.